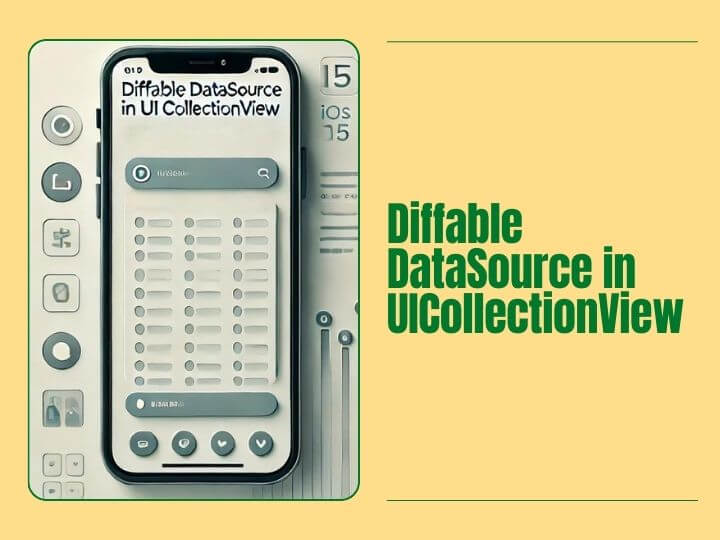
In this tutorial, you’ll learn how to implement Diffable DataSource in UICollectionView and UITableView. Diffable DataSource was introduced in iOS 13. It is the new way to manage DataSource for UICollectionView and UITableView that is more efficient and performant that the traditional approach.
What is Diffable DataSource?
The Diffable DataSource is an improvement over the legacy DataSource in UIKit for UITableView and UICollectionView. Diffable DataSource make it easier to update the contents of a UICollectionView or UITableView without having to reload the entire view.
As per my opinion The biggest advantage is you don’t need main thread to update your datasource. You can even update it from background thread, and the another one it removes data inconsistency errors like, in old age when you want to delete some cell, add some more and move some with single event than it was a nightmare. But with help of Diffable DataSource you can achieve it very easily.
There is basically 3 steps to implement Diffable DataSource.
- Create DataSource
- Create/Update Snapshot
- Apply Snapshot
Create DataSource
This is the first step where you configure your UICollectionView. Here is the code for the same.
private var dataSource: UICollectionViewDiffableDataSource<Section, Item>!
In the above code, the Section
must confirm the Hashable
protocol. Which will represent Sections of UICollectionView. The Item also must confirm the Hashable
protocol. Which will represent Items of UICollectionView. This should be at your ViewController’s property. Later on, you need it to modify.
dataSource = UICollectionViewDiffableDataSource<Section, Item>(collectionView: collectionView) { collectionView, indexPath, itemIdentifier in
let cell = collectionView.dequeueReusableCell(withReuseIdentifier: "cell", for: indexPath)!
// Configure here
return cell
}
Create/Update Snapshot
To create snapshot here is the code.
var snapshot = NSDiffableDataSourceSnapshot<Section, Item>()
snapshot.appendSections([Section.main])
snapshot.appendItems([item1, item2, item3])
Take a look on the following code to update snapshot.
var snapshot = dataSource.snapshot()
snapshot.deleteSections([Section.toDelete])
snapshot.insertSections([Section.latest], beforeSection: Section.main)
snapshot.reloadSections([Section.updated])
snapshot.insertItems([item4], afterItem: item2)
snapshot.deleteItems([item3])
snapshot.reloadItems([item2])
Apply Snapshot to Diffable DataSource
Here is the code to apply snapshot to update UICollectionView after creating or updating Snapshot.
dataSource.apply(snapshot, animatingDifferences: true)
By now you should be able to implement Diffable DataSource in your own iOS apps. This will make your applications more efficient and performant. It will also give you more control over how your data is displayed. You can read more about Diffable DataSource on Apple Developer Documentation
You can also take a look on Property Wrapper guide to write more maintainable code using Swift.